In today’s fast-paced digital landscape, businesses are increasingly adopting Software as a Service (SaaS) models for their applications. A multitenant architecture serves as a crucial component of these models, allowing a single instance of an application to efficiently serve multiple clients while keeping their data isolated and secure. This blog will guide you through the intricacies of creating a multitenant system using Django, PostgreSQL, and a NoSQL database, providing you with a strong foundation for building scalable SaaS applications.
Understanding Multitenancy
What is Multitenancy? Multitenancy refers to an architecture where a single instance of a software application serves multiple tenants (clients). Each tenant operates in a shared environment while maintaining separate and secure access to their data. This model is widely used in SaaS applications due to its cost efficiency, ease of maintenance, and scalability.
Benefits of Multitenancy:
- Cost Efficiency: By sharing resources, businesses can reduce operational costs.
- Simplified Maintenance: Updates and maintenance are performed on a single instance, ensuring all tenants receive the latest features simultaneously.
- Scalability: Easily add new tenants without significant changes to the application architecture.
Why Choose Django for Multitenancy?
Django is a high-level Python web framework known for its ease of use and rapid development capabilities. It comes with many built-in features, such as an ORM (Object-Relational Mapping) system, authentication, and an admin interface, making it an ideal choice for developing a multitenant SaaS application.
Key Advantages of Django:
- Robust Security: Built-in security features help protect against common threats like SQL injection and cross-site scripting.
- Rapid Development: Django's emphasis on reusability and “don’t repeat yourself” (DRY) principles speeds up the development process.
- Scalability: Designed to handle high traffic loads, Django is a great choice for applications expecting significant growth.
Project Setup: Step-by-Step
- Create a Django Project
Start by creating a new Django project using the following command:
bashCopy codedjango-admin startproject my_multitenant_app
- Install Required Packages
To facilitate multitenancy, install necessary packages, including PostgreSQL support and a tenant schema management package:
bashCopy codepip install django psycopg2-binary django-tenant-schemas
- Configure PostgreSQL
Set up PostgreSQL as your primary database. Each tenant will have its schema within the same database, ensuring data separation. You can create a database with the following command in your PostgreSQL prompt:
sqlCopy codeCREATE DATABASE my_multitenant_db;
- Set Up Tenant Schemas Configure your Django application to support tenant schemas. In your Django settings, specify the database routing and add middleware to manage tenant identification.
Incorporating NoSQL
In addition to PostgreSQL for structured data, incorporating a NoSQL database like MongoDB can help manage unstructured data effectively. This dual-database approach allows for flexibility in data handling and scalability.
Advantages of NoSQL:
- Schema Flexibility: NoSQL databases allow for a more flexible schema, accommodating unstructured data.
- High Performance: Designed for high-volume transactions, NoSQL databases can scale horizontally to handle increased loads.
Basic Architecture Overview
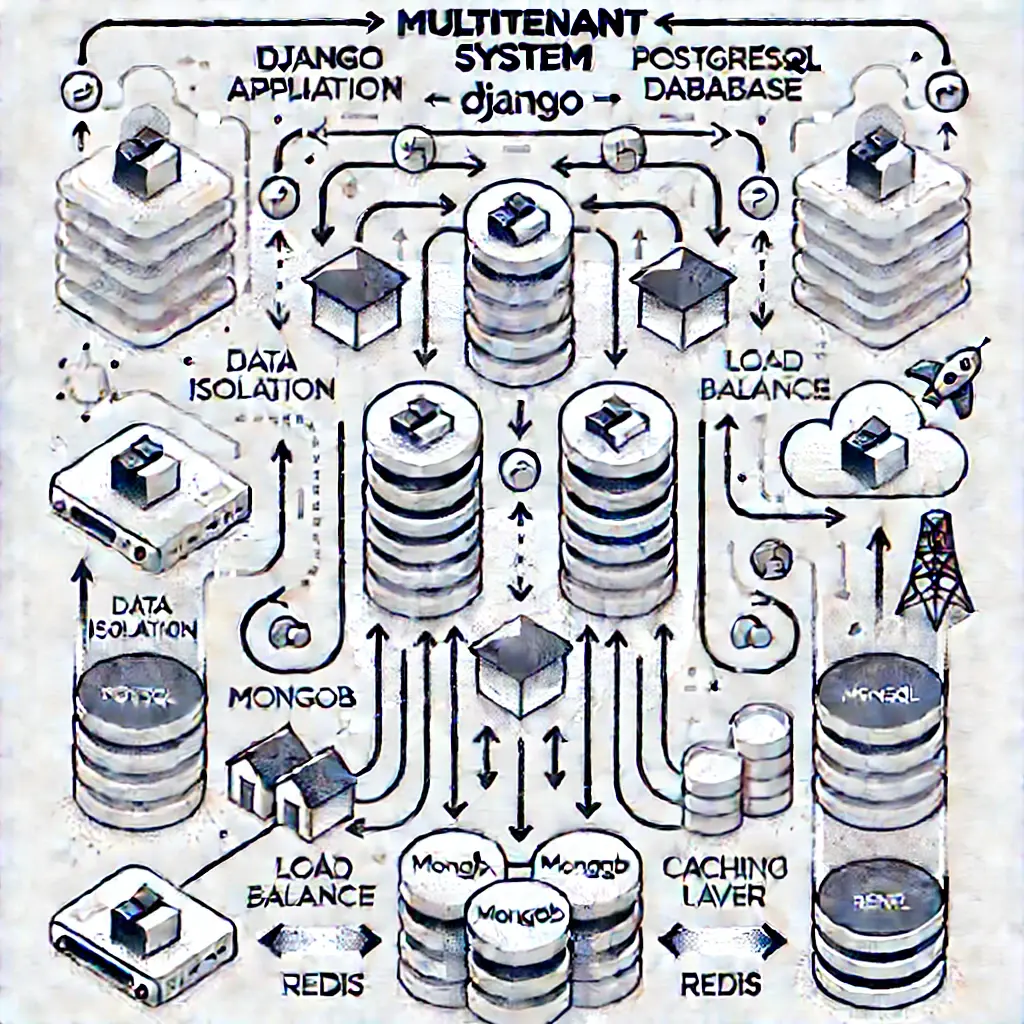
The architecture for a multitenant system built with Django, PostgreSQL, and NoSQL typically consists of:
- Django Application: The core application handling all user requests and business logic.
- PostgreSQL Database: Used for structured tenant data, where each tenant has a separate schema. Data isolation is crucial for ensuring compliance and security.
- NoSQL Database (MongoDB): For managing unstructured or semi-structured data, providing flexibility to the application.
- Load Balancer: A load balancer distributes incoming requests across multiple application instances, enhancing performance and reliability.
- Caching Layer (Redis): A caching layer, such as Redis, optimizes performance by caching frequently accessed data, reducing database load and response times.
Implementation Details
- Creating a Tenant Model
Create a model to define tenant-specific information, such as the tenant name and schema. For example:
pythonCopy codefrom django.db import models class Tenant(models.Model): name = models.CharField(max_length=255) schema_name = models.CharField(max_length=255, unique=True)
- Routing Requests Implement middleware to route requests to the appropriate tenant based on the subdomain or request header. This ensures that users access their specific tenant environment.
- Data Migration Migrate existing data to the new multitenant system. Use scripts to copy tenant data into the appropriate schemas within the PostgreSQL database and relevant collections in the NoSQL database.
- Testing and Validation Conduct extensive testing to validate data isolation and application performance under load. Ensure that tenants cannot access each other's data and that the application scales effectively.
Conclusion
Building a multitenant system using Django, PostgreSQL, and NoSQL is a strategic approach to developing SaaS applications that require scalability and data isolation. This architecture not only enhances security and compliance but also provides the flexibility to adapt to varying client needs.
By leveraging the strengths of each technology, you can create robust applications that meet the demands of multiple clients efficiently. As you embark on your journey to create a multitenant SaaS application, remember to consider the specific needs of your target audience, ensuring that your system is both user-friendly and highly functional.
Building a Multitenant System with Django, PostgreSQL, and NoSQL: A Comprehensive Guide